the best books for developers
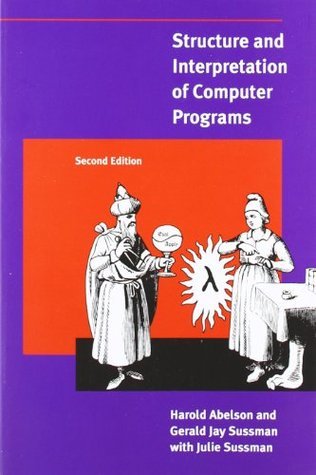
Topics: Algorithms, Data Structures, Functional Programming, Object-Oriented Programming, Compilers and Interpreters, Computer Architecture, Machine Code, Operating Systems, Networking, Artificial Intelligence, Security, Cryptography
By Harold Abelson, Gerald Jay Sussman, Julie Sussman
9780262510875
"Structure and Interpretation of Computer Programs" is like a magical journey into the heart of programming. Written by Harold Abelson, Gerald Jay Sussman, and Julie Sussman, this book is a treasure trove of insights for anyone who wants to become a coding wizard.
First, it takes you by the hand and introduces you to the world of programming using Scheme, a wonderfully elegant and powerful language. You'll learn the basics, like variables, data types, and control structures, but this book goes way beyond that.
The authors dive into the nitty-gritty of procedures and recursion, teaching you how to solve problems by breaking them down into smaller, more manageable pieces. It's like discovering the secret recipe for writing efficient and elegant code.
But that's just the beginning. You'll explore the art of abstraction, where you'll learn how to design your own data structures and create abstract representations of complex systems. It's like learning how to build your own LEGO set for solving any problem.
The book also delves into the realm of streams and delayed evaluation, showing you how to work with infinite data structures and create programs that can handle infinite streams of information. It's like learning how to manipulate time with your code.
Then, there's the magical world of metacircular evaluators. You'll build your own interpreter for Scheme, gaining a deep understanding of how programming languages work under the hood. It's like peeking behind the curtain of the programming universe.
As if that's not enough, the book explores concurrent programming, logic programming, and even explores the world of declarative programming with a dash of AI.
Throughout this journey, you'll discover the joy of thinking like a computer scientist. You'll gain the skills to tackle complex problems, design elegant solutions, and write code that's not just functional but truly beautiful.
So, if you're ready to embark on an adventure into the heart and soul of programming, "Structure and Interpretation of Computer Programs" is your magical guidebook. It's more than just a book; it's a portal to a world where you can create, innovate, and master the art of computer science.
Chapter 1: Building Abstractions with Procedures
In this foundational chapter, the authors introduce the concept of procedures as a means of abstraction in programming. They emphasize the importance of decomposing problems into smaller, manageable procedures and explore the fundamental principles of computer programming.
Chapter 2: Building Abstractions with Data
Chapter 2 delves into data abstraction, demonstrating how to create compound data objects and build abstract data types. The authors introduce pairs, lists, and sequences as fundamental data structures, enabling readers to work with structured data.
Chapter 3: Modularity, Objects, and State
This chapter explores the concept of modularity in programming and introduces the idea of state and mutable data. It covers the use of objects as a means of encapsulating state and behavior, providing insights into object-oriented programming.
Chapter 4: Metalinguistic Abstraction
Chapter 4 takes a deep dive into metalinguistic abstraction, where readers learn how to manipulate and extend the programming language itself. It introduces the concept of metacircular evaluators and lays the foundation for understanding the inner workings of interpreters.
Chapter 5: Computing with Register Machines
This chapter explores the world of register machines and low-level programming. It presents the idea of a register machine as a universal computational model and helps readers understand how high-level programming languages are implemented at a lower level.
Chapter 6: A Lisp Interpreter in Scheme
Chapter 6 builds on the previous chapters' concepts and guides readers through the creation of a Scheme interpreter. It provides practical experience in writing a metacircular evaluator and understanding the evaluation process of programming languages.
Chapter 7: The Metacircular Evaluator
In this chapter, the authors continue their exploration of metacircular evaluators. They introduce an extended version of the evaluator and delve into the details of lexical scoping and environments.
Chapter 8: Higher-Order Procedures
Chapter 8 introduces the concept of higher-order procedures, which can take procedures as arguments and return procedures as values. It explores powerful programming techniques enabled by higher-order functions, including map, filter, and reduce.
Chapter 9: Formulating Abstractions with Higher-Order Procedures
This chapter builds on the previous one, emphasizing the importance of creating abstractions with higher-order procedures. It covers the development of abstract data types and explores techniques for designing elegant and efficient programs.
Chapter 10: Modeling with Mutable Data
Chapter 10 explores the use of mutable data structures and models in programming. It discusses how to design programs that involve changing data over time, including simulation and modeling.
Chapter 11: Concurrency
Concurrency takes center stage in this chapter, as the authors introduce the challenges and techniques of concurrent programming. They discuss processes, message-passing systems, and synchronization primitives.
Chapter 12: Streams
Chapter 12 delves into the world of streams, which are sequences that can be infinite or delayed. The authors explore how to work with streams and use them for modeling and solving various problems efficiently.
Chapter 13: Metalinguistic Abstraction
This chapter revisits metalinguistic abstraction, focusing on the development of interpreters and compilers. It discusses the creation of domain-specific languages and the principles of language design.
Chapter 14: The Scheme Metacircular Evaluator
Chapter 14 extends the exploration of metacircular evaluators, presenting an implementation of the Scheme programming language within itself. It offers a deep dive into the design and implementation of interpreters and compilers.
Chapter 15: Logic Programming
The final chapter introduces logic programming and explores the principles of symbolic reasoning and unification. It provides a glimpse into the world of declarative programming and its applications in artificial intelligence.